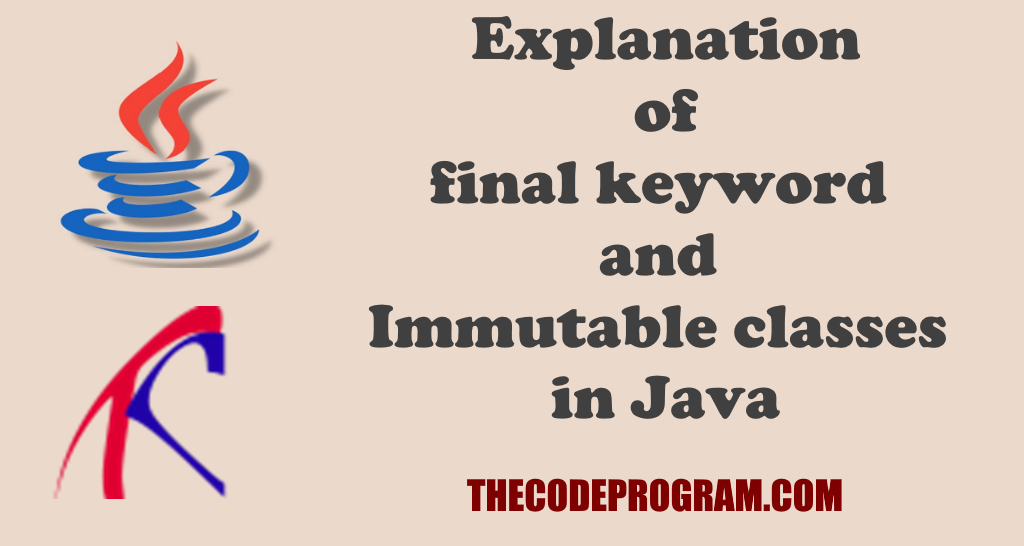
Explanation of final keyword and Immutable classes in Java
Hello everyone, in this article we are going to talk about final keyword and Immutable classes in Java programming language. We will make examples for better understanding.Let's get started.
final keyword is using for declaring the member as constant. We can assign values only one time to final entity declarations. final keyword can be used for variables, classes and functions.
As we mentioned above we can not assign a value at second time to a final variable. It means we can not reinitialize the final variable.
Below code block you will a simple exampe
import java.util.*;
public class Main {
public static void main(String[] args) {
final String name = "Burak Hamdi TUFAN";
System.out.println(name);
name = "Thecodeprogram.com";
System.out.println(name);
}
}
Compiler will throw below error:
Main.java:7: error: cannot assign a value to final variable name
name = "Thecodeprogram.com";
^
1 error
In this code block we try to reinitialze the final varialbe and compiler throw the error that specify we can not assign a new value to final variable.
As we mentioned above we can not derive new classes from final classes. It means we can not extend the final classes.
Below you will see an example.
final class Aircraft{
public static void printAC(){
System.out.println("Aircraft is Boeing...");
}
}
public class Main extends Aircraft {
public static void main(String[] args) {
printAC();
}
}
Compiler will throw below error
Aircraft.java:9: error: cannot inherit from final Aircraft
public class Main extends Aircraft {
^
1 error
error: compilation failed
As you can see above we tried to extend a final class and compiler did not allow us to extend a final class.
As we mentioned above we can not override the final functions in derived classes.
Below you will see an example.
import java.util.*;
public class Aircraft{
public final void printAC(String type){
System.out.println("Aircraft is " + type);
}
}
public class Boeing extends Aircraft{
public void printAC(String type){
System.out.println("Aircraft is " + type);
}
}
public class Main extends Aircraft {
public static void main(String[] args) {
Boeing b = new Boeing();
b.printAC("Boeing");
}
}
Compiler output will be like below:
Aircraft.java:10: error: printAC(String) in Boeing cannot override printAC(String) in Aircraft
public void printAC(String type){
^
overridden method is final
1 error
error: compilation failed
As you can see above we derived a new class from another class and we tried to override the final method within parent class. And compiler did not allow us to override a final method.
Until here we have seen the final declaration. From now on let's take a look at the Immutable classes.
Immutable class is a class that we initialise and then we can not change its content after initialisation. As you now in java there are built-in immutable classes exist like String, BigDecimal etc...
How to create an immutable class?
public class Main {
public static void main(String[] args) {
Aircraft ac = new Aircraft("Boeing", "777-300ER", 1999, 14523);
System.out.println(ac.getBrand() + '\n' + ac.getModel() + '\n' + ac.getYear() + '\n' + ac.getFlightCycle());
}
}
public final class Aircraft{
private final String brand;
private final String model;
private final int year;
private final int flightCycle;
Aircraft(String brand, String model, int year, int flightCycle){
this.brand = brand;
this.model = model;
this.year = year;
this.flightCycle = flightCycle;
}
public String getBrand(){ return new String(this.brand); }
public String getModel(){ return new String(this.model); }
public int getYear(){ return new Integer(this.year); }
public int getFlightCycle(){ return new Integer(this.flightCycle); }
}
Program outpu will be like below.
Boeing
777-300ER
1999
14523
Final look, When should we use final keyword:
final declerations are increasing the performance of program because they are being cached. finals let the compiler to optimize the code. As a best practice for final variable declerations we should use CAPS characters.
That is all in this article.
Burak Hamdi TUFAN
Comments