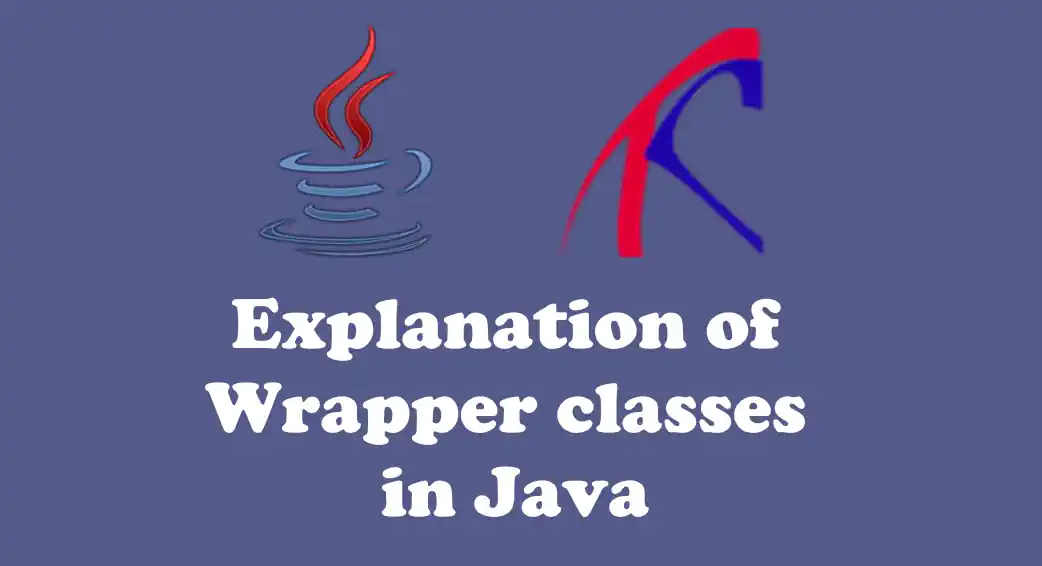
Explanation of Wrapper classes in Java
Hello everyone, in this article we are going to talk about the wrapper classes which are wrapping the primitive types that we discussed before in Java programming language.Let's begin.
In Java, data types are very important to keep and process the data. In Java we have two data types: primitives and reference types. Primitives are char, double int and etc..., and reference data types are object classes interfaces etc....
Wrapper classes are the classes to wrap the primitive types and offers useful methods to work with these primitive data types in object oriented programming model. We can think wraper classes as a bridge between primitive types and object oriented world.
In Java below list you can see the wrapper classes list.
Benefits of Wrapper Classes
Below you can see some benefits of wrapper classes:We can directly initialise with constructors of wrapper classes
Integer myInteger = new Integer(198);
Double myDouble = new Double(3.14);
Boolean myBoolean = new Boolean(false);
Static Factory Methods
Integer myInteger = Integer.valueOf(198);
Double myDouble = Double.valueOf(3.14);
Boolean myBoolean = Boolean.valueOf(false);
Autoboxing and Unboxing
Java provides the direct conversation between primitive types and their wrapper classes.
Below you can see the example of Autoboxing and Unboxing
// Autoboxing
Integer myInteger = 198;
Double myDouble = 3.14;
Boolean myBoolean = true;
// Unboxing
int primInt = myInteger.intValue();
double primDouble = myDouble.doubleValue();
boolean primBool = myBoolean.booleanValue();
That is all for wrapper classes.
Burak Hamdi TUFAN
Comments